Part 3 - Using The @EJB Annotation To Provide Dependency Injection Support In The Web Tier And Consuming The Services Provided By Their Backing EJBs
For this last part of the tutorial I am going to demonstrate how to use Wicket in an EE container environment such as Glassfish. wicket-contrib-javaee is a wicket module that provides integration through Java EE 5 resource injection. With wicket-contrib-javaee you can use the following 3 annotations in your wicket pages:- @EJB
- @PersistenceUnit
- @Resource
In particular, I will demonstrate how to use the @EJB annotation for dependency injection of the CustomerFacade ejb that was developed in Part 2 of this tutorial.
Adding wicket-contrib-javaee-1.1.jar
Begin by downloading the wicket-contrib-javaee-1.1.jar which can be found here on SOURCEFORGE.NET. Once you have downloaded the jar and saved it locally it then needs to be added to the WicketEETutorial1-war module:
- Right click the Libraries node and select Add Jar/Folder. This will open the Add Jar/Folder window.
- Navigate to the location where you saved the wicket-contrib-javaee-1.1.jar file and select it. Make sure that the Copy To Libraries Folder option is selected.
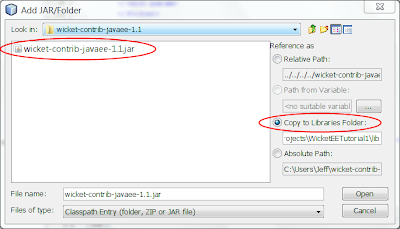
- Click Open and Netbeans will add the jar file to the module.
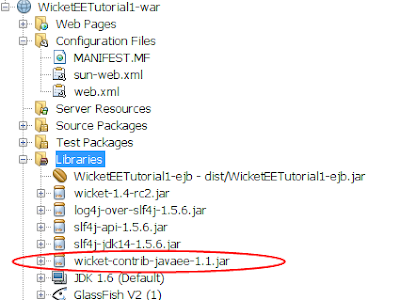
Now we need to add the wicket-ioc jar to the module. wicket-ioc.jar is included with the wicket distribution which we downloaded in Part 1 of this tutorial.
Adding wicket-ioc jar
- Right click the Libraries node and select Add Jar/Folder. This will open the Add Jar/Folder window.
- Navigate to the location where you saved the wicket distribution that we downloaded in Part 1 of this tutorial. In the lib folder select the wicket-ioc jar file. Make sure that the Copy To Libraries Folder option is selected.
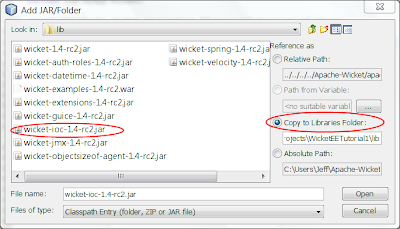
- Click Open and Netbeans will add the jar file to the module.
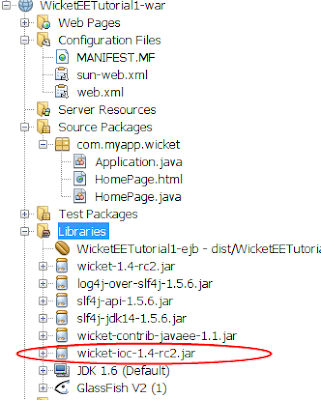
The wicket-ioc jar has a dependency on cglib jar so we need to include that in the module as well.
Adding cglib jar
The cglib jar is hosted on SourceForge and you can download it from here. Once you have downloaded the jar and saved it locally it then needs to be added to the WicketEETutorial1-war module:
- Right click the Libraries node and select Add Jar/Folder. This will open the Add Jar/Folder window.
- Navigate to the location where you saved the cglib jar file and select it. Make sure that the Copy To Libraries Folder option is selected.
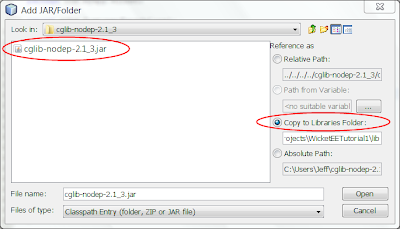
- Click Open and Netbeans will add the jar file to the module.
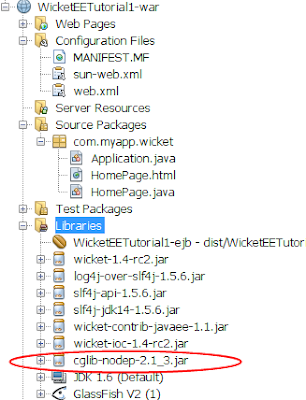
The web.xml file needs to be modified to include a reference to the CustomerFacade ejb.
Adding A Reference In web.xml To CustomerFacade EJB
In the Projects pane expand the Configuration Files node and double click the web.xml file to open it in the editor and click on the References tab.
- Expand the EJB References panel and click the Add button. This will open the Add EJB Reference window.
- Enter WicketEETutorial1-ejb/CustomerFacade for the EJB Reference Name.
- Select Session for the EJB Type.
- Select Local for Interface Type.
- Enter ejbs.CustomerFacadeLocal for the Local Interface.
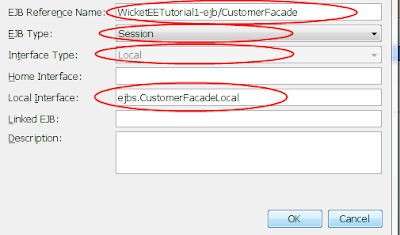
- Click the OK button to save the changes to the web.xml file.
Now we need to override the init method in Application.java.
Modifying Application.java
Open the Application.java file and add the following code:
@Override
protected void init() {
super.init();
addComponentInstantiationListener((IComponentInstantiationListener)
new JavaEEComponentInjector(this));
}
Right click in the editor and select Fix Imports to add the needed import statements.
Application.java file should now look like the following:
Now we need to modify HomePage.java where we wiill include the @EJB annotation for the CustomerFacade ejb and consume its services.
Adding The @EJB Annotation
Open HomePage.java in the editor and add the following to the HomePage class definition
:
@EJB(name="WicketEETutorial1-ejb/CustomerFacade")
private CustomerFacadeLocal customerEjb;
*** Note that the name we are providing for the @EJB annotation is the same one we used when we added the EJB reference to the web.xml file.
Now everything is in place for us to actually consume the data access services that are provided by the CustomerFacade ejb so lets add some more code to the HomePage constructor to get a list of Customer entities and get a count of how many entities there are in the list.
Consuming The Data Access Services Provided By The CustomerFacade ejb
Modify the HomePage constructor so that it looks exactly like the following:
public HomePage() {
add(new Label("sayhello","Hello, World!"));
List
customers = customerEjb.findAll();
int count = customers.size();
add(new Label("customercount",String.valueOf(count)));
}
Right click the editor and select Fix Imports to add the needed import statements. Your HomePage.java file should now look like the following;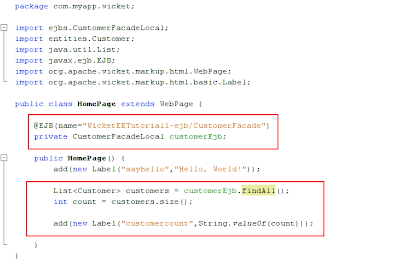
Open HomePage.html in the editor and modify its markup so that it looks exactly like the following;
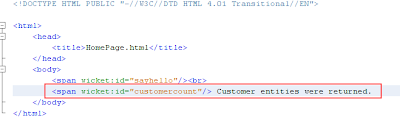
We have added a Wicket component that will display the number of Customer entities in the list of Customers returned from calling the findAll method of the CustomerFacade ejb.
We are now ready to build and run our application.
Running Our Application
Rick click the WicketEETutorial1 node in the Projects pane and select Run to build and run the application. Your browser should open and render the following;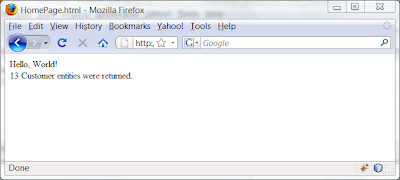
As you can see from the above image, we were able to obtain a reference to the CustomerFacade ejb via the use of the @EJB annotation and call the findAll method which the CustomerFacade ejb exposes. findAll returned a list of Customer entities and from the list we were able to obtain a count of Customer entities.
Summary
Netbeans greatly simplifies the whole process of generating the Enterprise Application's modules. Netbeans wizards generate a lot of code that we would otherwise have to hand code ourselves. To a very large degree Netbeans trivializes Java EE5 applications allowing us to focus on the domain issues of the application and not its plumbing and wiring.
Who said Java EE was too heavy and complex? I hope this tutorial demonstrated that neither is true.
With the addition of the wicket-contrib-javaee jar file, Wicket applications gain the ability to use Java EE5 annotations that allow it to take full advantage of services esxposed in enterprise java beans when running in a Java EE5 container such as the one Glassfish provides.
All in all, very cool, isn't it? And easy, too!And how cool is it to be able to use Wicket as the web framework in a Java EE application and have it be able to consume services exposed through ejbs using standard Java EE annotations?
Though this application was trivial by design so as to keep it simple for demonstration purposes, the principles demonstrated here will work for applications of much greater size and complexity.
Conclusion
This concludes the tutorial and I hope you had as much fun following along as I had presenting it. Please feel free to provide your comments. I look forward to hearing from you.
Great post Jeff!
ReplyDeleteAre the jars mentioned in the blog available in Maven repositories somewhere ?
Does using Maven somehow fix the problem of auto-deploy-on-save not working in NetBeans with Wicket applications?
ReplyDeleteI moved a wicket application from Eclipse to NetBeans - it does not use Maven. I deploy to GlassFish.
Unfortunately, when I change the .html files, they are not deployed on save. Only if I do a manual deploy on the project (which cannot be bound to a shortcut key :(
Thanks for any insight on working around that efficiency-killer.
Hi jskovzoftcorpdk,
ReplyDeleteThis is one of 2 known issue, both of which I had reported to the Wicket plugin author. The other issue is that the context menu opened when right clicking a non .java file in a java package will not allow the "safe" removal of the file from the project.
As you have discovered, you can manually deploy. When you right click on the project tab in the project pane you can select Deploy and force the issue. This is a work around until this is addressed formally by the Netbeans development community.
Unfortunately, there doesn't seem to be a workaround for removing a non .java file from a wicket project but all is not lost if you don't mind renaming files directly outside of Netbeans.
I hope this helps.
Jeff
Hi Arun,
ReplyDeleteAll the libraries used are available via Maven repositories and are 'findable' using the search facility built into Netbeans's Maven support.
You can search Maven repositories by right clicking on the project's Libraries node in the Projects pane and from the context menu select Add Dependency which will open the Add Dependency window where you can use the Query input field to search for the libraries.
Jeff
Many thanks for this great tutorial. I am convinced that NetBeans + EJB + Wicket is a sophisticated but simplifying and productive development environment.
ReplyDeleteIt will hopefully only take a short time until the issue that you are referring to is resolved:
http://www.netbeans.org/issues/show_bug.cgi?id=145666
I found another one that is more serious:
https://glassfish.dev.java.net/issues/show_bug.cgi?id=9107
@EJB breaks with Wicket Serialization
My suggestion is that interested developers add themselves to the cc list of these bugs and vote for them.
I know that the development community is interested in this type of feedback to set their priorities.
Bernard,
ReplyDeleteThank you for your kind words and your suggestion that interested developers add themselves to the cc lists for Wicket related bugs. Regarding the serialization and @EJB issue, if I can duplicate the problem I will post an article here with the details.
Jeff
Bernard,
ReplyDeleteI downloaded the test case for the issue that supposedly breaks @EJB in Wicket EE applications. After examining the jars included and not included in the WAR I believe the issuer of this bug report didn't know that the wicket-contrib-javaee-1.1.jar and wicket-ioc jar files are required and that the application also needs to make some configuration calls when it initializes. Therefore I believe this is not a valid issue. This 3 part article explains everything that is required to make Wicket and the @EJB annotation work. Please follow the examples given.
Please remember that Wicket is not currently inherently supported on Java EE application servers thus requiring some glue (wicket-contrib-javaee-1.1.jar and wicket-ioc jar) to make it all work.
Jeff
Jeff,
ReplyDeleteThanks for taking the time to examine the test case.
The inclusion of wicket-contrib-javaee-1.1.jar and the initialization code are prerequisites. The dependency on wicket-contrib-javaee-1.1 is not coded in the test case, but wicket-contrib-javaee-1.1 is actually at the core of the whole issue.
The issue is currently recorded against three different systems. Status in all is open and unchallenged. This means that wicket-contrib-javaee-1.1 cannot be used in the context that your tutorial is based on.
Wicket project lead Igor Vaynberg has reproduced the issue: https://issues.apache.org/jira/browse/WICKET-2416
I have of course notified the author of wicket-contrib-javaee-1.1 with http://wicketstuff.org/jira/browse/JAVAEE-5
Jeff, I share your enthusiasm about Wicket + Java EE + GlassFish + NetBeans.
Therefore I would highly appreciate if you could find a way to support the resolution of this critical bug in a key software component that connects Wicket with Java EE / GlassFish, for example by voting for it, or even better, by solving it.
Bernard
Bernard and all,
ReplyDeletePlease see my latest article. In it I address this issue.
Jeff
Bernard,
ReplyDeleteI do not get any errors or warnings when running this tutorial's application.
What errors do you get when you run the tutorial applications? Can you post the errors and the wicket log contents here? Other useful information to post would be the OS your glassfish server is running under, the versions of eclipselink/jpa, wicket and glassfish. Thanks.
Sincerely,
Jeff
Great! Thanks a lot for this post, it just saved me hours!
ReplyDeleteThank you very much for this post. I have tried to make JPA and JTA work with Wicket for several days without a result. I found this tutorial and introduced the whole EJB and it works. Thanks.
ReplyDelete